[TOC]
## 函数调用
- call的使用与import有点类似
- import只能调用优先级高的文件
- 而call插件独立的, call可以调用其他插件的call
外部插件目录: tphp/test
设置文件/html/plugins/tphp/test/base/page/call.php内容如下
```
<?php
return new class {
public function index($str = '')
{
return "这里是tphp/test插件目录: {$str}";
}
};
```
当前插件目录: tphp/demo
设置文件/html/plugins/tphp/demo/base/say/hello/call.php内容如下
```
<?php
return new class {
/*
* 默认访问路径,只要是public就可以访问到
* 也可以用 public static function index(){}
*/
public function index($str1 = '', $str2 = '')
{
return "sayHello: {$str1} {$str2}";
}
};
```
设置文件/html/plugins/tphp/demo/html/www/base/call/_init.php内容如下
```
<?php
return function () {
$info = [];
// . 和 / 效果是一样的
$info['a1'] = $this->plu->call('say.hello', 'Hello', 'world');
$info['a2'] = $this->plu->call('say/hello', 'Hello', 'TPHP');
// 以下访问也是等效的
$info['b1'] = plu()->call('say.hello', 'Hello', 'plu1');
$info['b2'] = plu('tphp.demo')->call('say.hello', 'Hello', 'plu2');
$info['b3'] = $this->plu->call('say.hello==tphp.demo', 'Hello', 'plu3'); // 或者 say.hello==demo
// 调用 tphp/test 插件函数
$info['c1'] = plu('tphp.test')->call('page', 'Hello TPHP');
$info['c2'] = $this->plu->call('page==tphp.test', 'Hello TPHP');
// 强 "==" 和弱 "=" 的区别
$info['d1'] = $this->plu->call('page', 'Hello TPHP'); // 获取不到数据
// 弱匹配:优先匹配tphp.test2,如果不存在其他插件是否有匹配,效率比较低,也容易出错,一般不使用
$info['d2'] = $this->plu->call('page=tphp.test2', 'Hello TPHP'); // 会匹配到tphp.test
// 强匹配: 只会匹配tphp.test2插件中的函数
$info['d3'] = $this->plu->call('page==tphp.test2', 'Hello TPHP'); // 无法匹配
dump($info);
};
```
打开网址[http://plu.tphp.com/base/call](http://plu.tphp.com/base/call)获得如下效果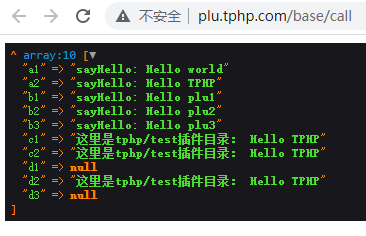
设置文件/html/plugins/tphp/demo/base/say/test/call.php内容如下
```
<?php
return new class {
// 或者 public $plu;
public static $plu;
private $num = 0;
public static function index()
{
dump(self::$plu);
}
public static function test($str1 = '', $str2 = '')
{
dump(self::$plu->call('say.hello', $str1, $str2));
return "<div>test方法</div>";
}
public function setNum($num = 0)
{
$this->num = $num;
}
public function addNum()
{
$this->num ++;
}
public function getNum()
{
return $this->num;
}
};
```
设置文件/html/plugins/tphp/demo/html/www/base/call/test/_init.php内容如下
```
<?php
return function () {
// 访问index方法
$this->plu->call('say.test');
// 或者
$this->plu->call('say.test:index');
// 访问test方法
echo $this->plu->call('say.test:test', 'Say', 'Test');
// 设置数字
$this->plu->call('say.test:setNum', 10);
// 数字 + 1
$this->plu->call('say.test:addNum');
// 获取数据值:11
dump($this->plu->call('say.test:getNum'));
// 获取数据值:0 会重新 new say/test类
// 每调用一次 callNew 都会 new 一个 class
dump($this->plu->callNew('say.test:getNum'));
// 获取数据值:11, 不会受到 callNew 影响
dump($this->plu->call('say.test:getNum'));
};
```
打开网址[http://plu.tphp.com/base/call/test](http://plu.tphp.com/base/call/test)获得如下效果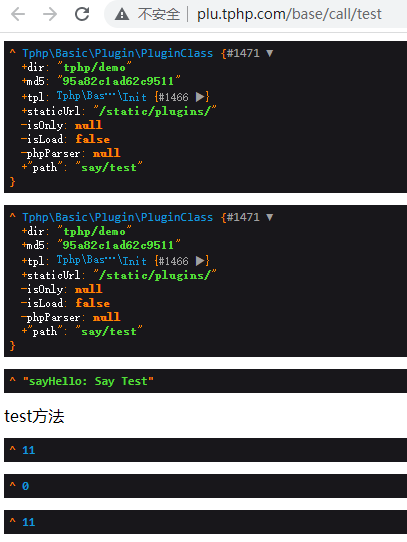
## caller 配置
- 可以在call.php中配置data.php、_init.php等文件
- 一个call.php文件就足够实现很多文件要处理的事情
设置文件/html/plugins/tphp/demo/html/www/base/caller/data.php内容如下
```
<?php
return [
'plu' => [
'caller' => 'caller'
]
];
```
设置文件/html/plugins/tphp/demo/html/www/base/caller/_init.php内容如下
```
<?php
return function () {
dump("Init From _init.php");
};
```
设置文件/html/plugins/tphp/demo/base/caller/call.php内容如下
```
<?php
return new class {
/**
* 等同于 data.php 文件
* @return array
*/
public function data()
{
return [
"type" => "sql",
"config" => [
"table" => "area",
"field" => [
"id",
"title"
],
"limit" => 3
]
];
}
/**
* 等同于 _init.php 文件
*/
public function _init()
{
dump("Init From call.php");
}
/**
* 等同于 src.php 文件
* @param $data
* @return mixed
*/
public function src($data)
{
dump($data);
return $data;
}
/**
* 等同于 ini.php 文件
* @return array
*/
public function ini()
{
return [
"#SQL" => [
"MyId" => [
["set", "MyId: _[id]_"]
]
]
];
}
/**
* 等同于 set.php 文件
* @param $data
* @return mixed
*/
public function set($data)
{
dump($data);
return $data;
}
};
```
打开网址[http://plu.tphp.com/base/caller](http://plu.tphp.com/base/caller)获得如下效果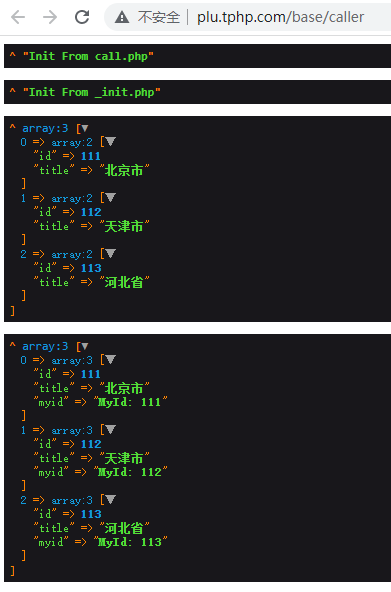